1.4 - Introduction to WebGL¶
What is WebGL?¶
Why learn WebGL? Very simply, it is the only cross-platform solution for producing real-time, 3D computer graphics we have.
What about Direct3D?
Direct3D is a proprietary graphics system made by Microsoft. Using Direct3D restricts the execution of your graphics to Microsoft based devices.
What about OpenGL?
OpenGL is cross-platform and executes on Windows, Lunix, MacOS, etc., but OpenGL does not have a definitive way to interface to an operating system’s user interface. There is a code library that makes OpenGL cross-platform development possible called GLUT (or FreeGlut), but GLUT does not include the standard user interface elements (e.g., buttons, slider bars, input boxes, etc.) that most graphics applications tend to use.
WebGL is a JavaScript API (application programmer interface) that allows a programmer to use the graphics processing unit (GPU) of a computing device in a web browser window to render real-time 3D computer graphics. Basically WebGL is a software driver that gives you a single interface to all commonly used GPU’s. When you update hardware, such as purchase a new phone, your WebGL applications still work because the WebGL driver knows how to communicate with the new hardware. Given that hardware is outdated in only a few years, and that you don’t want to rewrite your graphics applications that often, using WebGL is a great win for software development.
WebGL is a subset of OpenGL. WebGL executes in all modern web browsers, including web browsers on tablets and phones. Therefore, when you learn WebGL, you are learning OpenGL for an environment that basically will execute on all modern computing devices. That is really cool!!!
What is a WebGL Program?¶
A typical web page that contains 3D computer graphics generated by a WebGL program is made up of these basic components:
- A HTML (Hypertext Markup Language) description of the web page which contains one or more canvas elements to render the 3D graphics.
- A CSS (Cascading Style Sheet) that describes how elements of the HTML document are formatted.
- A HTML canvas element in the web page that provides a rectangular area in which 3D computer graphics can be rendered.
- Graphical data that defines the 3D objects to be rendered, along with descriptions of the lights in the scene and the camera that defines the current view.
- JavaScript programs that:
- loads your graphics data (such as model descriptions),
- configures your graphical data,
- renders your graphical data, and
- responds to user events.
- WebGL Shader programs that perform critical parts of the graphical rendering.
All of these basic components initially reside on a web server. When a user (a client) requests a web page that contains 3D graphics, the data described above has to be transferred to the client’s computer, stored in correct places, and then processed by appropriate processors.
When a client requests a web page from a web server, the following sequence of events happen:
- The server sends the HTML description of the web page to the client and the client stores the web page in RAM.
- The client’s web browser (e.g., Chrome, Safari, Firefox) reads the HTML page description and requests any other documents that are referenced in the HTML code, including JavaScript code, CSS (cascading style sheets), images, data files, etc. All of these files are downloaded and stored in the client’s RAM.
- If there is JavaScript code associated with the page, it is
executed. For a page that contains 3D graphics, the JavaScript code
performs the following tasks:
- It might request that other data files be downloaded from the server (for example, the data that describes the 3D graphic objects to be rendered).
- It retrieves and initializes the WebGL graphics context associated with the HTML canvas element where the graphics will be rendered.
- It configures and copies any model data to the GPU’s memory. (Technically the data is copied to GPU vertex object buffers).
- It establishes “event handlers” that associate HTML events with JavaScript code. The code is executed when an event happens.
- Finally, the web page is rendered to a window in the browser and the web page waits for user events. Timer events can be set up to automatically generate events on a regular schedule, which can create animations of the 3D graphics.
To re-emphasize the “big picture,” the diagram below summarizes the above discussion. Note that the JavaScript code runs on the CPU(s) and the graphics rendering is done by the GPU(s). The JavaScript code is the “master”. It “runs the show” and tells the GPU(s) when and what to render. The GPU(s) execute the graphics pipeline and make real-time graphics possible because of their speed of rendering.
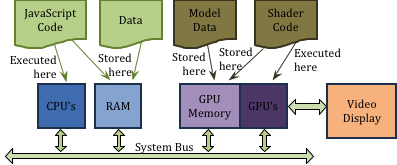
Where the different parts of a WebGL program are stored and executed
When a JavaScript event handler initiates the rendering of graphics into a HTML canvas element, it tells the GPU which object buffer(s) contain the data it wants to render and then issues the WebGL “draw” command. In a typical graphics program, the model data is copied to the GPU’s memory only once. The rendering is efficient and fast because the data is local to the GPU. If the transfer of data between RAM and the GPU’s memory is minimized, real-time graphics is possible in the browser.
A basic principle of all computer graphics is that expensive operations should only be done once. Throughout this textbook you will continually see the phrases “pre-processing step” and “rendering step”. The goal is to perform the “pre-processing step”, which is done by the CPU, only once. This allows the actual rendering that is done by the GPU to be as fast as possible.
All of the JavaScript code in this textbook that performs graphics rendering is divided into two separate parts:
- a setup (or pre-processing) part that is done once, and
- a rendering part that is performed each time an object is drawn.
Each lesson will make a clear distinction between pre-processing code and rendering code.
Tutorial Examples¶
A web page interface that allows you to view WebGL code, data, and the results of a WebGL rendering has been developed for this textbook and an example is shown below. The interface allows you to experiment with a WebGL program interactively. Remember, personal experimentation is critical to your learning! Pause when you come to the interactive examples and experiment!
The interface includes commands across the top of the WebGL example that allow for the following options:
- Re-start - If you edit the code displayed in the left window panes, you can click the “Re-start” button to update the WebGL program using your modified code and/or data. If you introduce errors in the WebGL program it will not execute properly. If this is the case, you should see error messages displayed in the output window pane below the canvas. In some cases, the errors will only be visible in the JavaScript console window. If you can’t resolve the errors that you introduced into the program, you can always start over by re-loading the entire web page. If text in an editor pane is highlighted with a light gray background, the text is “readonly” and can’t be modified. In the demo below you can edit the definition of the cone model, but only view the HTML code.
- Show checkboxes - Allows you to hide any of the three display panels in the WebGL interactive example. You will find it helpful to hide the canvas while you are studying and editing the editor panes.
- Download Files - Downloads all of the files that make up this WebGL program to your computer’s default download folder. These are the original files that created the WebGL program. The number of files can be large, depending on the complexity of the example. It is recommended that you empty your download folder before downloading a WebGL program so you don’t miss any important files. To use the code files in a stand-alone program the files will have to be organized appropriately in sub-folders. (More on this later.)
- Download Edited Files - Downloads a copy of the files you have edited in the editor panels. The downloaded copy will have any changes you have made to the text.
The interactive example below is shown here for you to familiarize yourself with the interface. All of the edit windows are read-only for this example. Simply examine the HTML, JavaScript, and model data to see what they look like. You can interact with the graphics in this specific example by clicking and dragging your mouse in the canvas window. You can also enable or disable the automatic animation using the checkbox below the canvas window. If you would like to see the results of the WebGL program as a separate, independent page, use the hyperlink at the bottom of the interactive example. Depending on your default browser settings, the WebGL program will open in a new window or a new tab.
An example of using WebGL to render a 3D animated object.
Animate
Download the files for the example above and then look in you computer’s download folder at the files that were created. This will help you understand what is required for a WebGL program.
Summary¶
This lesson explained how a web page that contains a WebGL program works. The details can be overwhelming and confusing, so please study this lesson one more time before going to the next lesson.
Glossary¶
- mastery learning
- learn a foundational topic completely before moving on to harder more advanced topics.
- web server
- a program that runs on a server computer and stores web pages and related files. When a client computer requests a web page, the web server sends the requested files to the client.
- web browser
- a program that runs on a client computer which allows a user to download, view, and interact with web pages. Commonly used web browsers are Google Chrome, Mozilla Firefox, Apple Safari, and Microsoft Internet Explorer.
- CPU
- central processing unit - the hardware that controls a computing device and performs all of its calculations.
- GPU
- graphic processing unit - the hardware that is optimized to produce 3D computer graphics.
- RAM
- random access memory - where data and programs are stored for CPU access and manipulation.
- GPU memory
- where data and programs are stored for GPU access and manipulation.
- pre-processing step
- manipulation of data that happens once.