2.2 - HTML and CSS¶
HTML (hypertext markup language) and CSS (cascading style sheets) are used to describe web pages. HTML describes the content of the page while CSS describes the formatting of individual elements. If a web page is designed with special care, the HTML code can remain static, while the CSS style sheets can change based on the target screen. For example, a web page might have very different formatting for a large desktop computer screen as compared to a mobile phone screen.
Tutorials¶
To build WebGL programs you need to understand the basics of HTML and CSS code; you don’t have to master all their intricate complexities.
Basic HTML and CSS can be learned from many on-line tutorials. Please use the tutorials at code academy to learn how to create basic HTML and CSS code.
STOP!
Please don’t proceed with this lesson until you have learned basic HTML and CSS.
Resources¶
These “cheat sheets” will be helpful to you:
This exhaustive cheat sheet for HTML 5 might be overwhelming, but useful at the same time:
If you want to experiment with HTML and CSS, https://jsfiddle.net/ is an excellent “sandbox” where you can immediately view the results of your HTML and CSS code.
HTML User Input¶
The main HTML element tag we will use for WebGL programs is the <input ... />
element which allows a user to specify values that can be used to control
a canvas 3D rendering. Here is a list of the standard input elements we will use
to create user interfaces:
input type | HTML code | Example rendering |
text | <input type="text" value="abc" /> |
|
number | <input type="number" value="10" min="1" max="20" step="0.5"/> |
|
range | <input type="range" value="15" min="10" max="20" step="0.1"/> |
|
button | <input type="button" value="click me" /> |
|
image | <input type="image" alt="some text" src="_images/login.png" width="50%" height="50%" /> |
|
checkbox | <input type="checkbox" checked="checked" /> <span>Example</span> |
Example |
radio | <input type="radio" name="my_radio" value="one" /> abc<br /> <input type="radio" name="my_radio" value="two" /> def<br /> <input type="radio" name="my_radio" value="three" /> ghi<br /> |
abc def ghi |
color | <input type="color" value="#FF0000" /> |
Each <input ... />
element will be assigned a unique ID so that it can be easily
accessed and modified as needed. For example: <input id="button1" type="button" value="click me" />
.
HTML Coding Standards¶
These coding standards were introduced in chapter 1, but please study them again now that you better understand HTML and CSS.
Rule | Correct Example(s) | Incorrect Example(s) |
Always declare the document type as the first line in your document. | <!doctype html> |
|
Use lower case element names. | <p>, <div> |
<P>, <Div> |
Close all elements. | <p>example</p> |
<p>example |
Close empty elements. | <br /> |
<br> |
Use lowercase attribute names. | <div class="..."> |
<div CLASS="..."> |
Quote all attribute values for consistency. | <div class="example"> |
<div class=example> |
Don’t use spaces around equal signs. | <div class="..."> |
<div class = "..."> |
Try to avoid code lines longer than 80 characters. | ||
For readability, add blank lines to separate large or logical code blocks. | ||
For readability, add 2 spaces of indentation for code inside a parent element. Do not use TAB characters. | <p>
Indented text.
</p>
|
<p>
Non-indented text
</p>
|
Always include a <html> , <head> and <body> tag. |
||
Include appropriate comments. | <!-- This is a comment --> |
|
Use simple syntax for linking style sheets. | <link rel="stylesheet"
href="styles.css"> |
|
Use simple syntax for loading external scripts. | <script src="myscript.js"> |
|
Use the same naming convention in HTML as JavaScript. | ||
Always use lower case file names. | my_file.txt |
MyFile.Txt |
Use consistent file name extensions. | .html, .css, .js,
.frag, .vert, .obj, .mtl |
.HTM, .text |
Always include the language and character encoding meta-data and the <title> element. |
A complete example:
1 2 3 4 5 6 7 8 9 10 | <!doctype html>
<html lang="en-US">
<head>
<meta charset="UTF-8">
<title>Example</title>
</head>
<body>
Page elements
</body>
</html>
|
Summary¶
As with all software development, when you design a web page you should start with a very simple HTML document and add complexity little by little.
Self-Assessments¶
-
Q-31: In a HTML document, which tag contains all of the page’s visible elements?
- <body>
- Correct, the body tag contains the page's visible elements.
- <head>
- Incorrect, the head tag contains meta-data about the page.
- <html>
- Incorrect, the html tag delimits the entire document.
- <title>
- Incorrect, the title tag gives a name to the page that is displayed in the browser's tab.
-
Q-32: Which HTML
- image
- Correct, <input type="image" ... > element is a button created from an image.
- number
- Incorrect, the input type number a user to pick a number from a range of possible values.
- range
- Incorrect, the input type range presents a slider bar that can be dragged to specify a number in a range.
- color
- Incorrect, the input type color presents a color picker in a separate, pop-up window.
<input>
type allows you to create a button from an image?
-
Q-33: A set of radio type
- Every radio button in a group has the same "name" attribute.
- Correct, the "name" attribute places a radio button in a unique group.
- Every radio button in a group has the same "value" attribute.
- Incorrect, the "value" attribute allows you to assign a unique value to the radio button.
- Every radio button in a group has the same "type" attribute.
- Incorrect, the "type" attribute is always "radio" for all radio buttons.
- Every radio button in a group has the same "step" attribute.
- Incorrect, radio buttons do not use the "step" attribute.
<input>
tags only allow one of the radio buttons to be
selected at one time. How does the browser know which radio buttons form a group?
-
Q-34: When should
- When the input values inside the <form> need to be sent back to the web server.
- Correct, <form>s tags group values that need to be sent back to the server.
- Always!
- Incorrect, use <form>s only when the input values need to be sent to the server.
- Never!
- Incorrect, use <form>s when the input values need to be sent to the server.
- When you need to arrange the input values in a particular layout.
- Incorrect, arrangement of input elements is done by normal HTML and CSS formatting.
<input>
tags be enclosed in a <form>
?
-
Q-35: Which of the following HTML code strings violate the coding standard? (Select all that apply.)
- <DIV>example</DIV>
- Correct, the <DIV> tag should be in lower case, such as <div>
- <input> type="number" value=50 />
- Correct, all attributes should be enclosed in quotes, such as <input> type="number" value="50" />
- <input type="image" src="MyImage.png" />
- Correct, all file names should be lower case, such as <input type="image" src="my_image.png" />
- <p>example</p>
- Incorrect, this HTML code meets the coding standard.
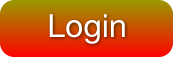